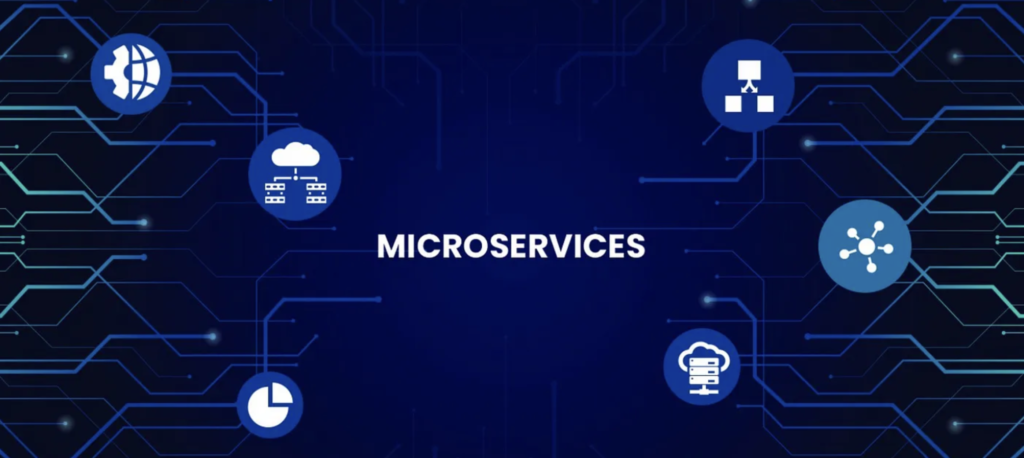
Microservices have revolutionized software architecture, offering scalability, maintainability, and independent deployment. But building a robust microservice architecture requires careful consideration of various design patterns. This guide dives deep into popular microservice design patterns, exploring their use cases, benefits, drawbacks, and providing real-world scenarios where they shine.
Why Microservices and Design Patterns?
Microservices address the limitations of monolithic applications by breaking them down into smaller, independent services. This distributed nature introduces new challenges like inter-service communication, data consistency, and fault tolerance. Design patterns provide proven solutions to these challenges, ensuring your microservice architecture is efficient, resilient, and scalable. For a deeper dive into the benefits and challenges of microservices, check out Martin Fowler’s seminal article on Microservices.
1. API Gateway Pattern: The Central Hub
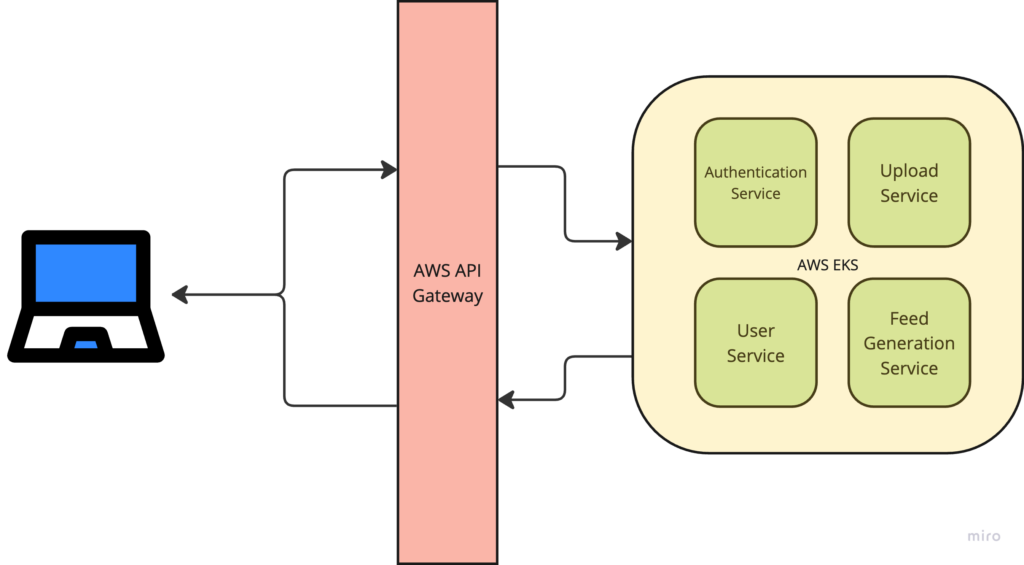
- Problem: Clients interacting with numerous microservices face complexity, tight coupling, and security vulnerabilities. Direct client-to-service communication increases latency and exposes internal services.
- Solution: An API Gateway acts as a single entry point for all client requests. It routes requests, aggregates responses, handles authentication and authorization, and can implement rate limiting and other cross-cutting concerns. Popular API Gateway implementations include Kong and cloud-based solutions like AWS API Gateway and Azure API Management.
- Use Cases:
- E-commerce platforms: Managing requests for product information, orders, and payments.
- Mobile applications: Aggregating data from multiple backend services for a seamless user experience.
- IoT platforms: Handling a high volume of requests from connected devices.
- Advantages:
- Simplified client interaction.
- Enhanced security.
- Improved performance through aggregation and caching.
- Disadvantages:
- Single point of failure.
- Potential performance bottleneck.
- Increased complexity.
2. Saga Pattern: Orchestrating Distributed Transactions
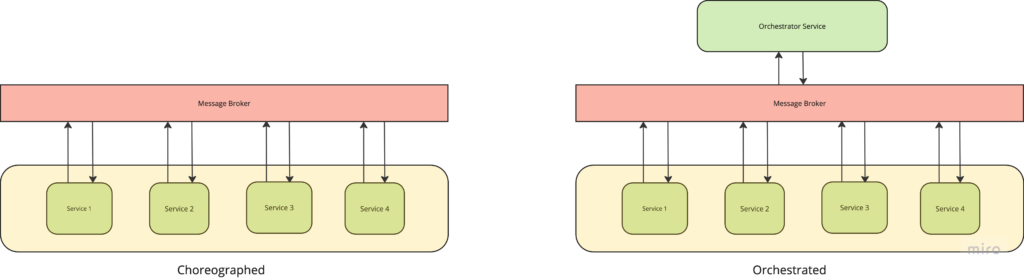
- Problem: Traditional ACID transactions aren’t suitable for distributed microservices. Maintaining data consistency across services requires a different approach.
- Solution: The Saga pattern manages distributed transactions as a sequence of local transactions. Each service completes its transaction and publishes an event that triggers the next service in the sequence. Compensating transactions handle failures by undoing previous steps. For a detailed explanation of the Saga pattern, see this article.
- Use Cases:
- Online ordering systems: Managing the order process across multiple services like inventory, payment, and shipping.
- Banking applications: Transferring funds between accounts involves multiple services.
- Advantages:
- Ensures eventual consistency.
- Avoids complex distributed transactions.
- Supports long-running transactions.
- Disadvantages:
- Complex implementation and debugging.
- Requires careful design of compensating transactions.
- Eventual consistency can lead to temporary inconsistencies.
3. Circuit Breaker Pattern: Preventing Cascading Failures
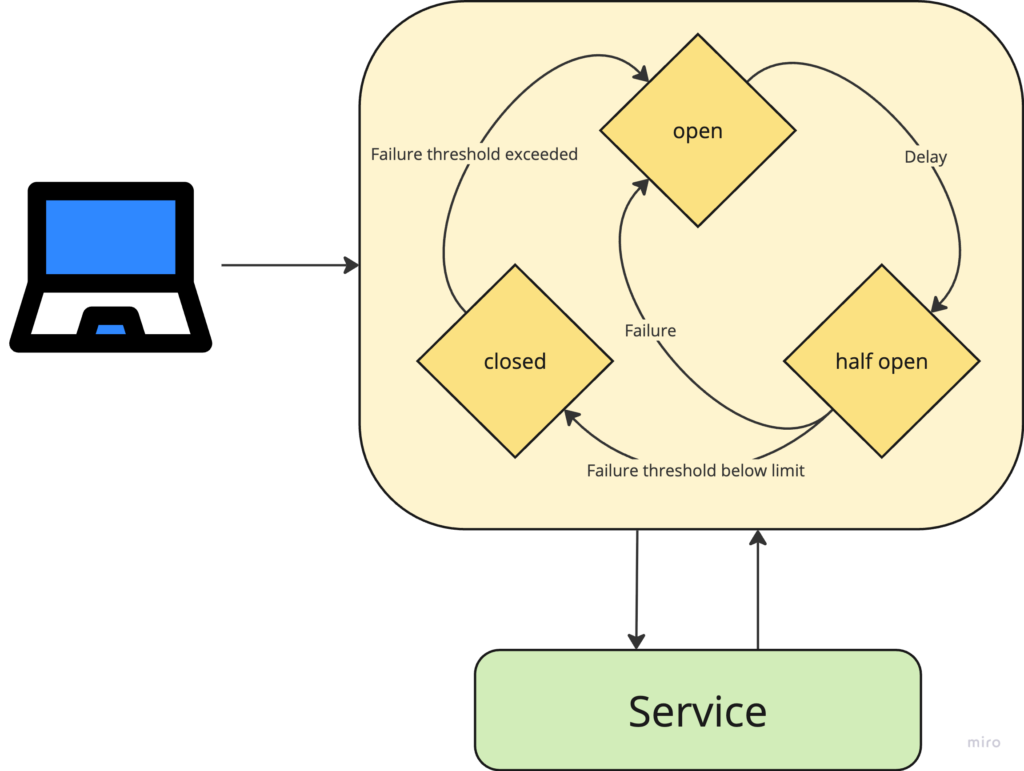
- Problem: A failing service can trigger a cascade of failures, bringing down the entire system. Constant retries to a failing service exacerbate the problem.
- Solution: A Circuit Breaker acts as a proxy to a service. It monitors the service’s health and trips the circuit if the failure rate exceeds a threshold. While the circuit is open, requests are short-circuited, preventing further calls to the failing service. After a timeout period, the circuit breaker allows a limited number of test requests to check if the service has recovered. Libraries like Hystrix and Polly provide Circuit Breaker implementations.
- Use Cases:
- Any application that relies on external services, especially those prone to instability.
- Microservices communicating over a network.
- Advantages:
- Prevents cascading failures.
- Improves system resilience.
- Reduces load on failing services.
- Disadvantages:
- Requires careful tuning of thresholds and timeouts.
- Can block legitimate requests if configured too aggressively.
- Adds complexity.
4. Event-Driven Architecture (Choreography & Orchestration): Asynchronous Communication
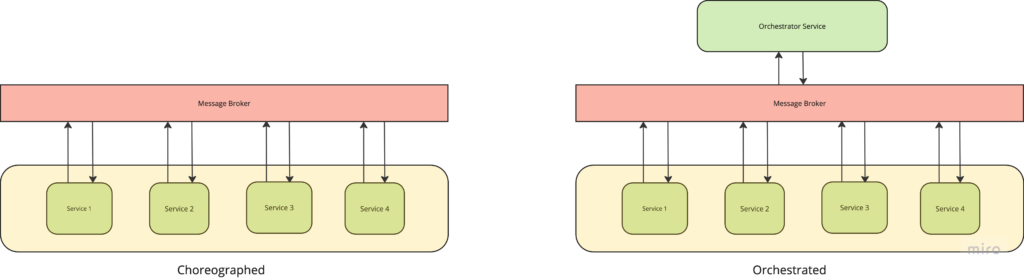
- Problem: Tight coupling between microservices through synchronous communication (like REST) can lead to performance bottlenecks and reduced flexibility.
- Solution: Services communicate asynchronously using events. In choreography, services publish events, and other services react to them. In orchestration, a central orchestrator manages the flow of events and service execution. Message brokers like RabbitMQ and Kafka are often used in event-driven architectures. Cloud providers also offer managed message queues like AWS SQS and Azure Service Bus.
- Use Cases:
- Real-time analytics: Processing streams of events from various sources.
- E-commerce order processing: Different services react to order creation events.
- Advantages:
- Loose coupling.
- Scalability and fault tolerance.
- Improved responsiveness.
- Disadvantages:
- Debugging can be challenging.
- Message ordering and duplication need to be handled.
- Orchestration can introduce a single point of failure if not designed carefully.
5. Strangler Fig Pattern: Migrating Monoliths
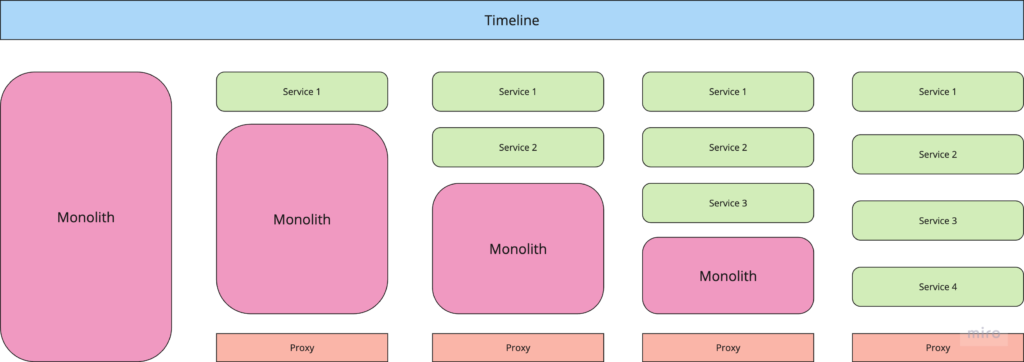
- Problem: Migrating a monolithic application to microservices is a complex and risky undertaking.
- Solution: The Strangler Fig pattern allows for gradual migration. New functionality is implemented as microservices, while existing functionality remains in the monolith. Traffic is gradually shifted to the new microservices until the monolith is “strangled” and can be decommissioned.
- Use Cases:
- Modernizing legacy applications.
- Incrementally migrating to a new technology stack.
- Advantages:
- Low-risk migration.
- No downtime required.
- Allows for testing microservices in a production environment.
- Disadvantages:
- Increased complexity in routing requests.
- Potential performance issues during migration.
6. Database Per Service Pattern: Data Isolation
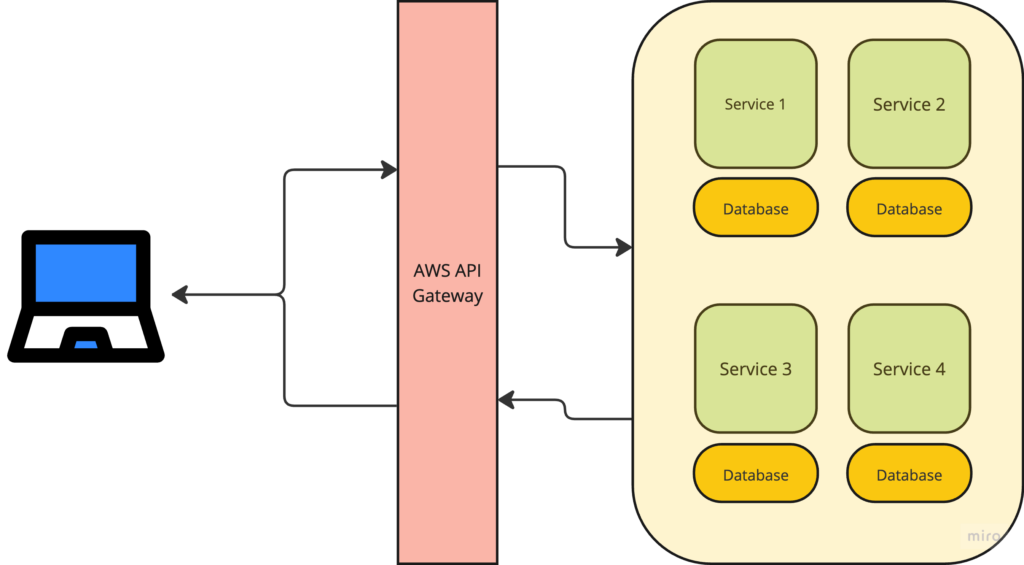
- Problem: Sharing a database between microservices creates tight coupling and reduces autonomy.
- Solution: Each microservice owns its database. Communication between services happens through APIs or events, not direct database access.
- Use Cases:
- Most microservice architectures benefit from this pattern.
- Essential for achieving true microservice independence.
- Advantages:
- Improved service autonomy.
- Independent schema evolution.
- Prevents data coupling.
- Disadvantages:
- Data duplication.
- Increased complexity.
- Eventual consistency.
7. CQRS (Command Query Responsibility Segregation): Optimizing Reads and Writes
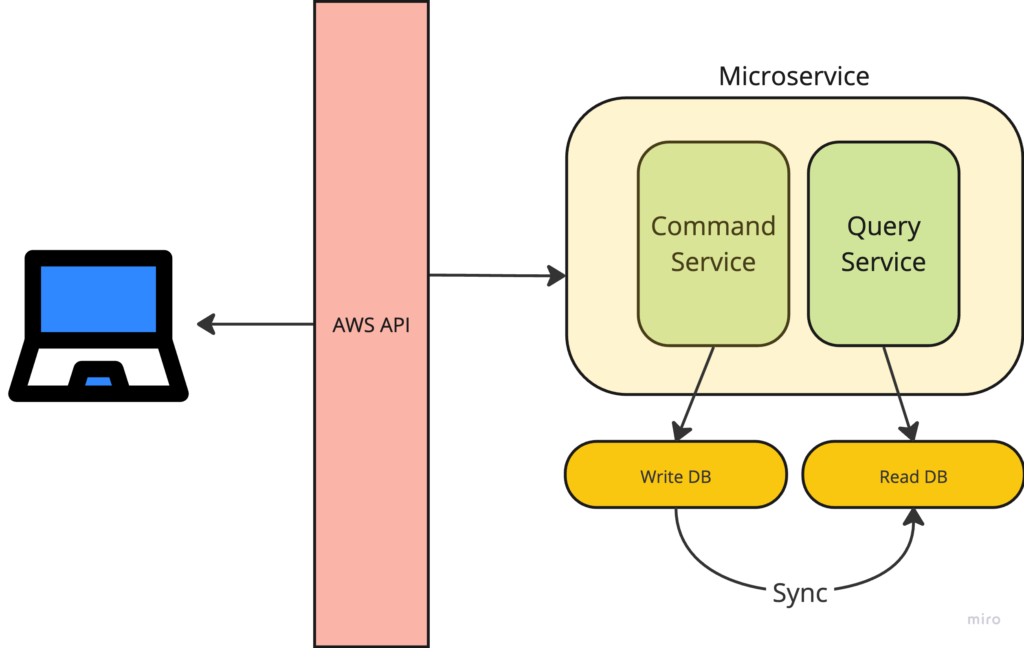
- Problem: A single database for both reads and writes can become a performance bottleneck, especially with complex queries.
- Solution: CQRS separates the read and write models. The write model handles commands (updates), while the read model maintains optimized views for queries.
- Use Cases:
- Applications with high read traffic and complex queries.
- Systems requiring event sourcing for auditability.
- Advantages:
- Improved read performance.
- Optimized write operations.
- Disadvantages:
- Increased complexity.
- Eventual consistency.
- More infrastructure overhead.
Best Practices
1. Idempotency
Ensure operations are idempotent so that retries don’t result in duplicate actions.
2. Event Versioning
Use versioning to manage event schema changes without breaking dependent services.
3. Dead Letter Queues (DLQs)
Handle unprocessable events by routing them to DLQs for later investigation.
4. Observability
Monitor distributed transactions using tools like Jaeger or Zipkin to trace event flows and identify bottlenecks.
5. Retry with Backoff
Implement exponential backoff strategies to avoid overwhelming downstream services during retries.
6. Compensation Mechanisms
Design compensating transactions to undo changes in case of failure.
Tools and Frameworks
Here are some popular tools and frameworks to implement distributed transactions:
- Message Brokers: Apache Kafka, RabbitMQ, Amazon SQS.
- Saga Frameworks: Temporal.io, Axon Framework, Camunda.
- Distributed Tracing: OpenTelemetry, Jaeger, Zipkin.
- Event Stores: EventStoreDB, Kafka.
Conclusion
Microservice design patterns are essential tools for building robust and scalable systems. Choosing the right patterns depends on your specific needs and context. Understanding the trade-offs of each pattern is crucial for successful microservice implementation. Don’t be afraid to experiment and adapt these patterns to fit your unique requirements. By carefully considering these patterns, you can unlock the full potential of microservices and create truly resilient and scalable applications.
Leave a Reply